Hi Reynaldo,
Please find below two solutions to handle this.
The first solution works without script with all dashboard Re-size modes including Responsive with template grids.
The second solution is the scripts, which works with Template Grids but not in Responsive mode.
I hope you find them useful:
First solution – Using Layer, Rectangle and labels:
-
You can create a new Layer, and then in the target cell of that layer, create a Rectangle which contains one or more labels, each representing one command, as shown below:
-
Add the script on Click event of each label, so it does what they are supposed to do.
-
Add ‘Change Layer’ Interaction to Click event of the labels as well, so it hides the layer containing the context menu after it is clicked:
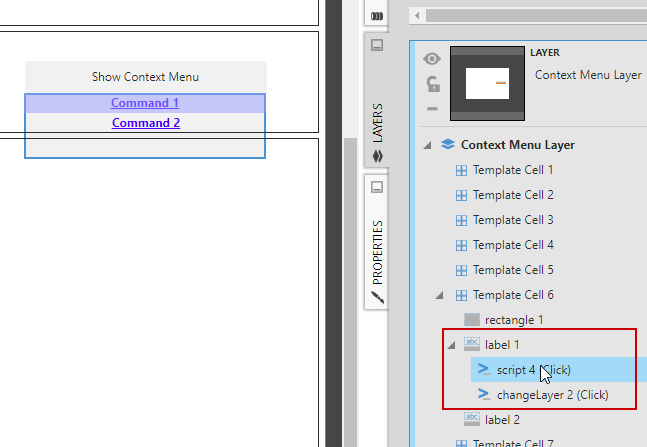
-
In the Click action of your button, create a Change Layer Interaction, so it shows the layer once this is clicked,
-
Hide This layer by default, by clicking on Eye icon as shown below:
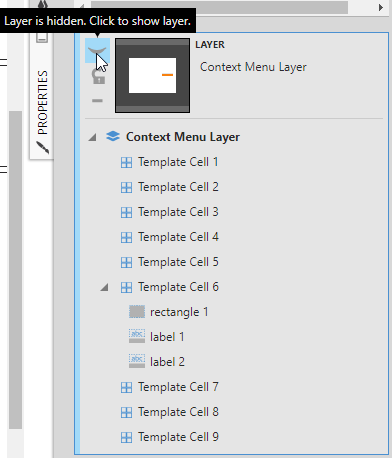
-
Switch to View mode and test it with different screen sizes.
-
Now, you may change properties of the Rectangle and Labels so it looks closer to a real Context Menu.
Second Solution (which works with Template Grid, but not in Responsive mode):
In the sample below, I have used Terrence’s sample code and some of your scripts to calculate the coordinates that the Context menu should be displayed.
This script is added to the Click action of a button that is placed in Cell 6 of a 3X3 template grid.
------------------------------------*********************************-------------------------------------------
debugger ;
// Calculate Coordinates (top and left)
var layerAdapters = this . parentView . layerAdapters ;
var layer = layerAdapters [ 0 ];
var screenWidth = layer . container . clientWidth ;
// Please change the templateCell index accordingly, (it should be the index of the cell that contains the button)
// Template cell index starts from 0 and is from left to right - top to bottom
var widthOfTemplateCell = this . parentView . templateCells [ 5 ]. width ;
var templateCellLeft = screenWidth ***** this . parentView . templateCells [ 5 ]. left ;
var screenLeft = (( screenWidth ***** widthOfTemplateCell ) ***** this . left ) + templateCellLeft ;
var heightOfTemplateCell = this . parentView . templateCells [ 5 ]. height ;
var screenHeight = layer . container . offsetHeight ;
var templateCellTop = screenHeight ***** this . parentView . templateCells [ 5 ]. top ;
var screenTop = (( screenHeight ***** heightOfTemplateCell ) ***** this . top ) + (( screenHeight ***** heightOfTemplateCell ) ***** this . height ) + templateCellTop ;
var coordinate = new dundas . controls . Coordinates ( screenLeft , screenTop , null , this . getService ( "CanvasService" ). canvas , null , this );
var commands = [];
// define new commands and push to the command
var parentMenuItem = new dundas . Command ({
caption : "Share" ,
categoryName : "Share" ,
action : function () {
// Do cool stuff here
}. bind ( this ),
subCommands : [
new dundas . Command ({
id : dundas . Utility . createGuid (),
caption : "PDF" ,
categoryName : "Export to PDF" ,
description : ‘Export to PDF’ ,
editModeOnly : false ,
action : function () {
var share = new dundas . controls . ExportDialog ( dundas . constants . STANDARD_PDF_EXPORT_PROVIDER_ID );
var sharePanel = share . show ();
}. bind ( this )
}),
]
});
commands . push ( parentMenuItem );
window . dundas . context . getService ( "ContextMenuService" ). showContextMenu ( commands , e . originalEvent , {
"getAdapter" : function () {
return this ;
}. bind ( this ),
"coordinate" : coordinate . convertCoordinateKind ( dundas . controls . CoordinateKind . PAGE , null , this . getService ( "CanvasService" ). canvas . getVision (). scale )});
----------------------------------------***********************----------------------------------------
I hope these help.